1. Introduction
Hi there! I am Delong, currently a second year student at National University of Singapore major in Computer Science.
I like building things from scratch. Since young, I like building LEGO. And ever after starting to programme, I fell in love with it and enjoy creating my own web app, phone app and software.
This project is part of a Software Engineering module (CS2103T) that I have taken. Together with my teammates, Keith, Zhuanyu, Yurou and Alvin, I would like to present Car park Finder to you.
2. Project Overview: Car park Finder
Car park finder is a desktop address book application to find HDB (Housing Development Board) car parks in Singapore. It allows you to work with a Command Line Interface (CLI) to display a list of car parks with the convenience of simply typing. If you use the computer frequently and commute by driving, you would find our application useful in helping you obtain various information about car parks.
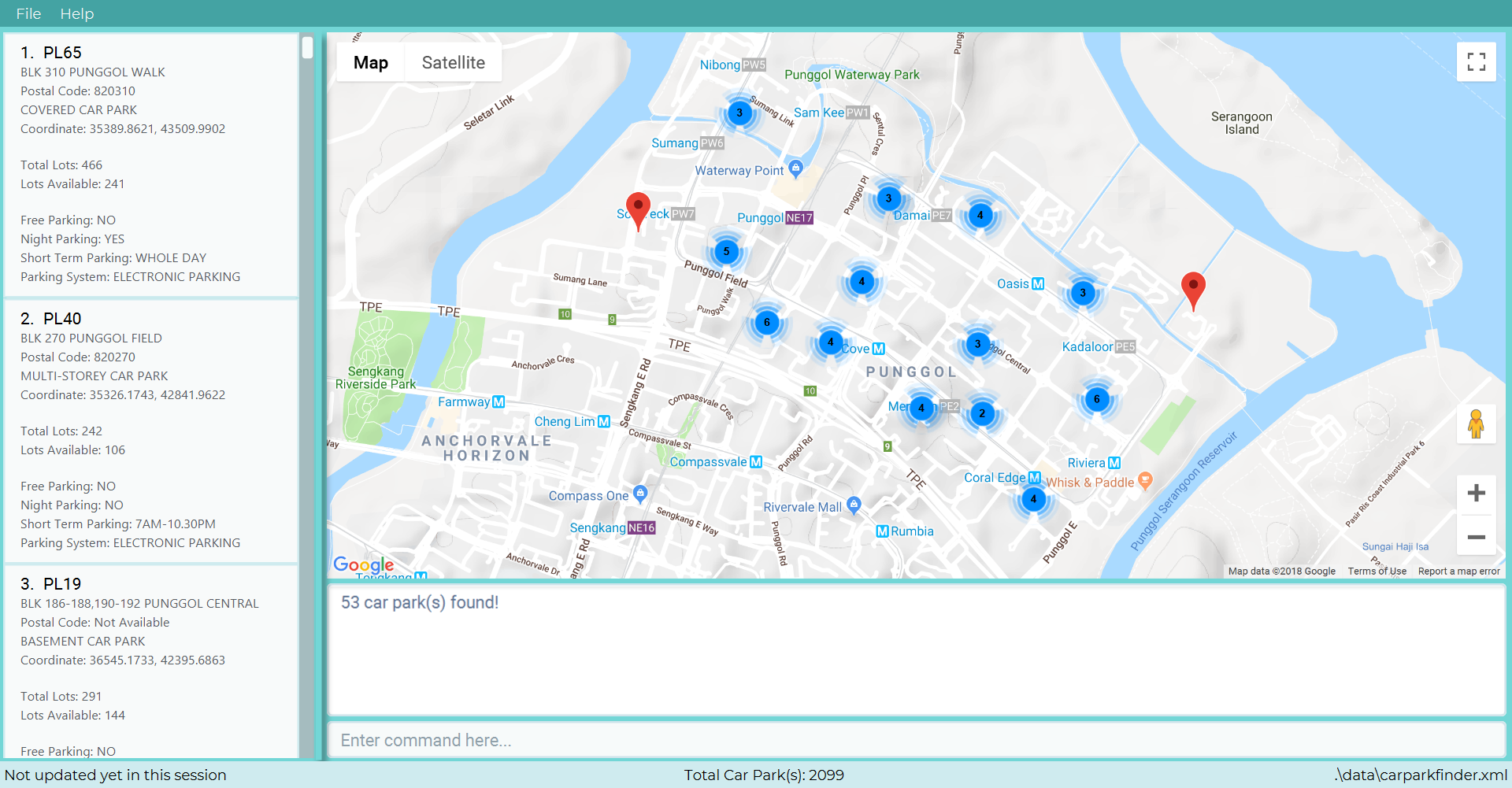
3. Summary of contributions
Here is the list of my contributions to this project, ranging from adding features to documentations:
-
Major enhancement: Add auto-complete feature to commands that require parameters.
-
What it does: It prompts the user the full format of a command.
-
Justification: It improves the product significantly because some of our commands can have up to three parameters. Memorising the full format is time-consuming.
-
Highlights: This enhancement allows the user to highlight the next parameter placeholder after one placeholder is filled up.
-
-
Minor enhancement: Add command abbreviation feature to most frequently used commands.
-
What it does: It allows the user to type less for the same functionality.
-
Justification: It improves the product significantly because command words can be long and typed wrongly easily.
-
Highlights: This enhancement is very intuitive and thus easy to use.
-
-
Code contributed: [Command Box], [Client Syntax], [Carpark Finder Parser], [Carpark Finder Parser Test].
-
Other contributions:
4. Contributions to the User Guide
Here is the list of my contributions to the User Guide. They showcase my ability to write documentation for end-users to understand easily. We are targeting car owners, e.g. taxi drivers.
4.1. Abbreviation: abbrev.
If you find the command words too long to type. Guess what? We have executable short form for each command in our command list.
You can find abbreviations of each command in the table that we provide to each section from 3.1 to 3.19.
However, there are ambiguous abbreviations that are not distinguishable between multiple commands.
Thus, you CANNOT use them: c , f , fi , h .
|
To see how useful it can be, please take a look at the examples below.
4.1.1. Example: Calculate Command
Step 1. Instead of entering calculate
into the Command Box, you can enter ca
instead.
Step 2. complete the rest of the fields with TJ39 SUN 7.00AM 9.00PM
, followed by pressing Enter to execute.
4.2. Autocomplete: Tab
If you forgotten how to type a command, do not worry! By typing in the first letter of the command in the Command Box and pressing the Tab key, it will display the full format of the command.
To see how useful it can be, please take a look at the examples below.
4.2.1. Example: Select Command
Step 1. Enter s
into the Command Box, followed by pressing Tab.
Your select
command will be completed.
Step 2. Substitute the selected field INDEX
with 1
, followed by pressing Enter to execute.
4.2.2. Example: Filter Command
Step 1. Enter fil
into the Command Box, followed by pressing Tab.
Your filter
command will be completed.
Step 2. Substitute the selected field DAY
with SUN
, followed by pressing Tab to move to the next field.
Step 3. Repeat step 2 until you substitute all the fields with actual value. (You can remove those fields you choose not to use). Then press Enter to execute.
Appendix A: Command Summary
This is the last section of the User Guide, but the most useful if you just want a list of commands to try. Refer to General Commands for general commands and Car Park Management for car park management. For more details on what each command does, please refer to [Features].
Command | Format | Example |
---|---|---|
Help |
help |
he |
List |
list |
l |
Undo |
undo |
u |
Redo |
redo |
r |
Clear |
clear |
cl |
History |
history |
hi |
Exit |
exit |
e |
Command | Format | Example |
---|---|---|
Query |
query |
q |
Select |
select INDEX |
s 2 |
Find |
find KEYWORD [MORE_KEYWORDS] |
fin punggol |
Filter |
filter FLAG/ PARAMETER [MORE_FLAG/ PARAMETER] |
fil f/ SUN 7.30AM 5.30PM ct/ SURFACE |
Calculate |
calculate DAY START_TIME END_TIME |
cal SUN 3.30PM 6.30PM |
Notify |
notify TIME_SECONDS |
n 60 |
5. Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
5.1. Architecture overview
This section explains the design architecture used by the entire system.
The .pptx files used to create diagrams in this document can be found in the diagrams folder. To update a diagram, modify the diagram in the pptx file, select the objects of the diagram, and choose Save as picture .
|
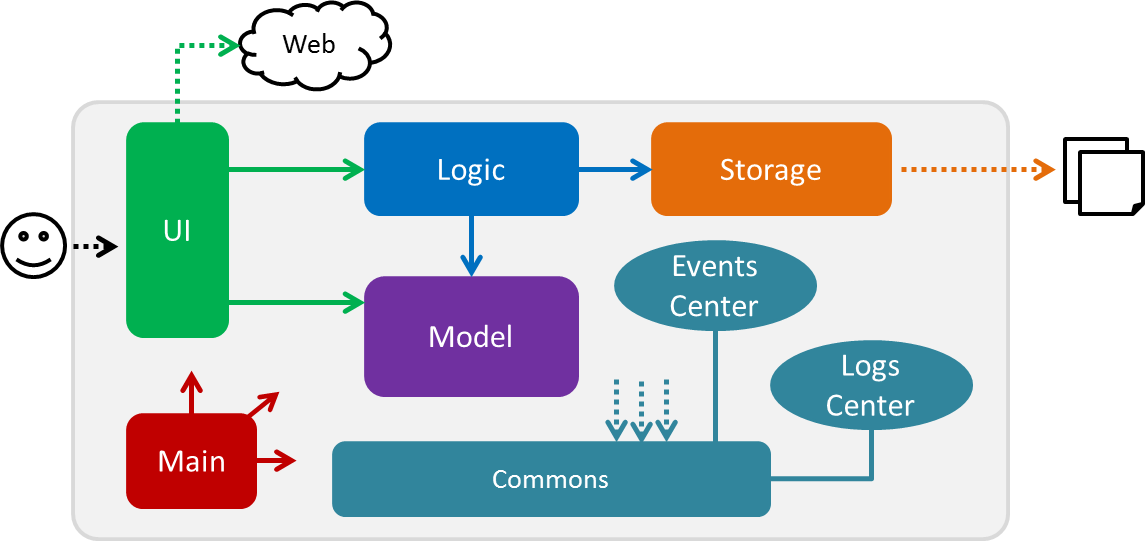
The architecture diagram given above explains the high-level design of the App,
which contains 6 architecture components
and
adopts an events-driven nature
.
5.2. Architecture components
This section will briefly introduce the function of each architecture components as well as common behaviours.
Component | Main Function |
---|---|
the starting point of the system, which encapsulates the other components. |
|
represents a collection of classes used by multiple components. |
|
contains the user interface classes used by the application. |
|
execute user commands, also known as the command executor. |
|
holds the data of the application in-memory. |
|
which allows reading and writing of data to the hard disk. |
Each of the User Interface, Logic, Model, Storage Components also: |
-
Defines its API in an
interface
with the same name as the Component. -
Exposes its functionality using a
{Component Name}Manager
class.
For example, the Logic
component (see the class diagram given below) defines it’s API in the Logic
interface and exposes its functionality using the LogicManager
class.
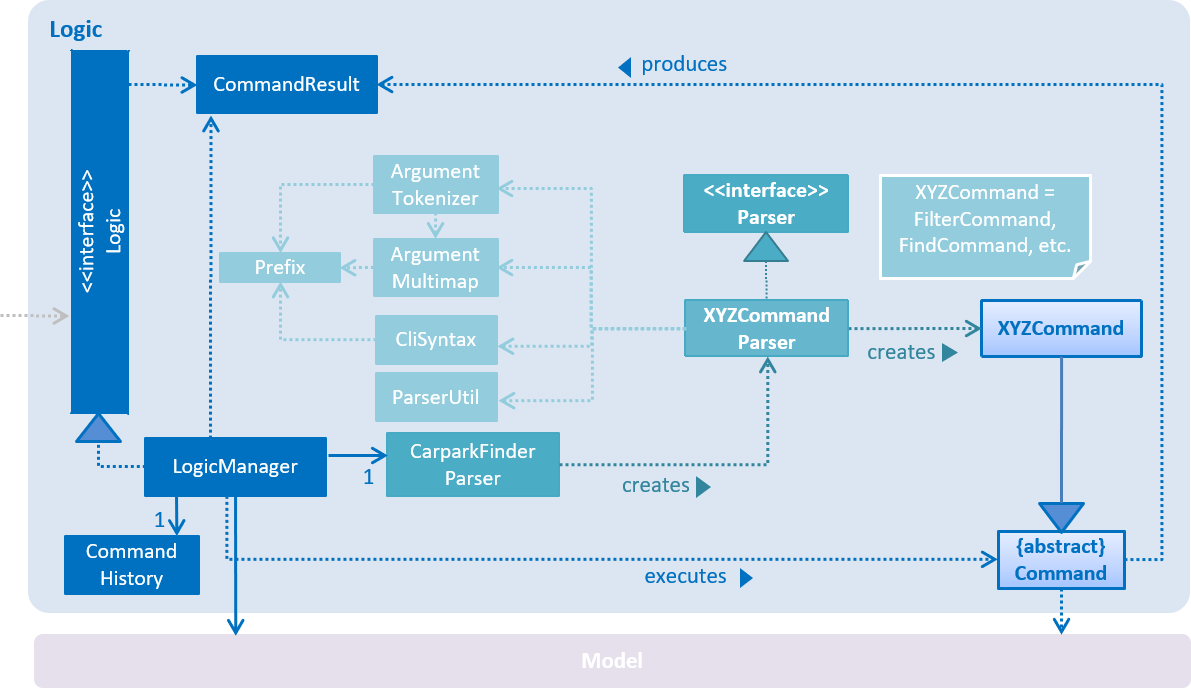
5.3. Main component
The Main
component consists of only one class, MainApp
. It is responsible for:
-
At app launch: initializes the components in the correct sequence, and connects them with one another.
-
At shut down: shuts down the components and invokes cleanup methods where necessary.
5.4. Commons component
The Commons
component consists of classes used by multiple other components. Two of these classes play important roles at the architectural level.
-
EventsCenter
: This class (written using Google’s Event Bus library) is used by components to communicate with other components using events (i.e. a form of Event Driven design) -
LogsCenter
: Used by many classes to write log messages to the App’s log file.
Classes used by multiple components are put in the seedu.carparkfinder.commons package. |
5.5. User interface component
The User Interface
(UI
) component consists of a MainWindow
that is made up of different parts.
The base class
Ui.java
uses JavaFx UI framework.
Please refer to the class diagram below for more details on how they are connected.
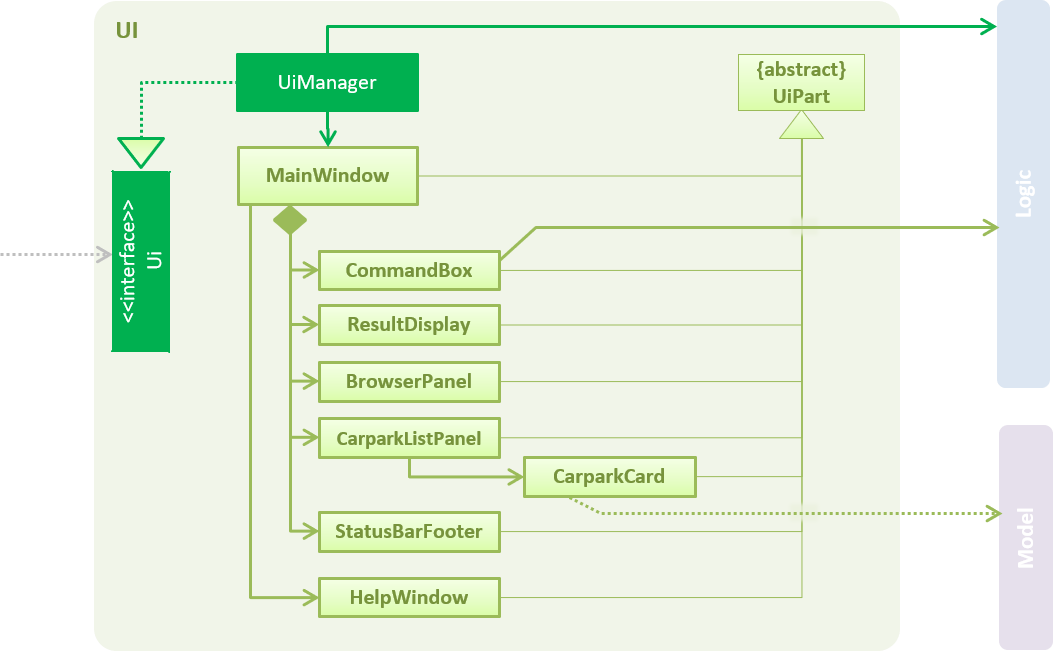
In general, this is the workflow of the UI
component:
-
Execute user commands using the
Logic
component. -
Bind itself to some data in the
Model
so that theUI
components can auto-update when data in theModel
change. -
Respond to events raised from various parts of the App and updates the
UI
components accordingly.
All UI
parts, including the MainWindow
, inherit from the abstract UiPart
class. The layout for each
component is defined in matching .fxml
files and can be found in the src/main/resources/view
folder.
For example, the layout of the MainWindow
is specified in MainWindow.fxml
.
5.6. Model component
The Model
component is managed by the ModelManager
that stores the data of Car Park Finder.
It does not depend on any of the three other components.
Model.java
is the base class.
Please refer to the class diagram below for more details.
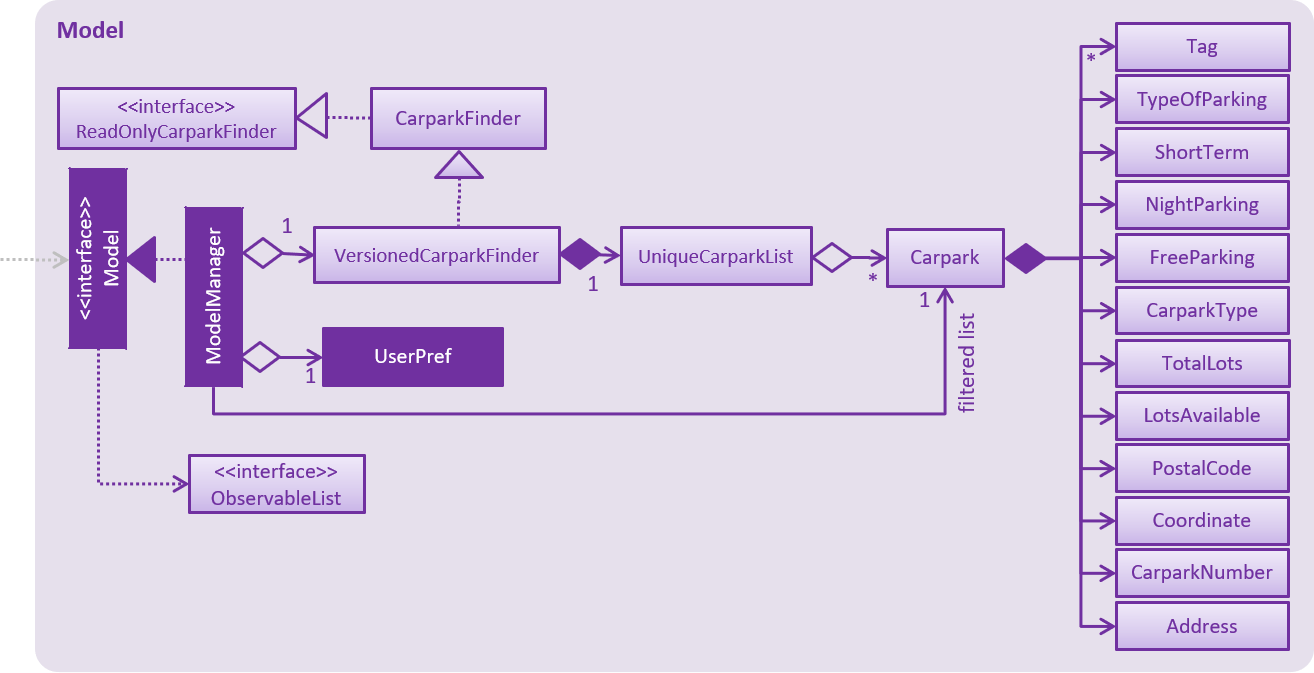
In general, this is the structure of the Model
Component:
-
The
ModelManager
extends theModel
Interface. -
It stores a
VersionedCarparkFinder
and aUserPref
object.-
The
UserPref
object represents the user’s preferences. -
The
VersionedCarparkFinder
contains acarparkFinderStateList
which is used to store multipleCarpark
objects.
-
-
The
ModelManager
also manages a filtered list ofCarpark
objects filtered from thecarparkFinderStateList
. -
The
Model component
exposes an unmodifiableObservableList<Carpark>
that can be 'observed' e.g. the UI can be bound to this list so that it automatically updates when data in the list changes.
As a OOP model, we can store a Tag list in Car Park Finder, which Carpark can reference.
This would allow Car Park Finder to only require one Tag object per unique Tag , instead of
each Carpark needing their own Tag object. An example of how such a model may look like is given below.
|
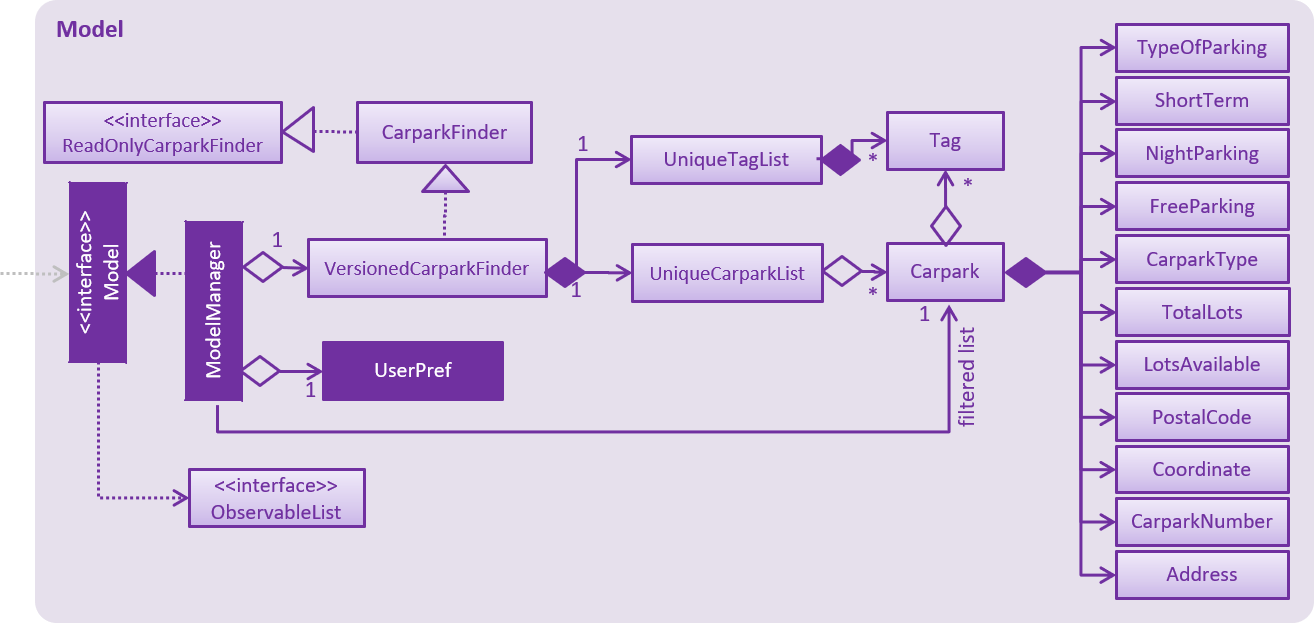
5.7. Storage component
The Storage
component, managed by the StorageManager
, serves as a backend storage for data of Car Park Finder.
Storage.java
is the base class.
Please refer to the class diagram below for more details on how they are connected.
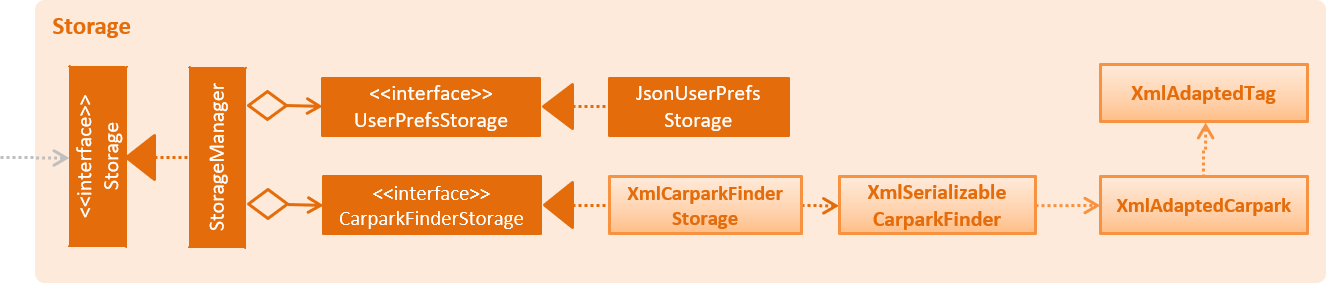
The Storage
component can perform the following functions:
-
save
UserPref
objects in json format and read it back. -
save the Car Park Finder data in xml format and read it back.
5.8. Abbreviation
The abbreviation feature reduces the amount of characters needed to type.
5.8.1. Overview
The abbreviation mechanism is facilitated by CarparkFinderParser
. It extends the cases
to allow command abbreviations to be parsed through parseCommand
as well.
5.8.2. Example
Given below is an example usage scenario and how the abbreviation mechanism behaves at each step.
Step 1. The user launches the application for the first time. The LogicManager
is initialized with an CarparkFinderParser
.
Step 2. The user executes c
command instead of calculate
. The Matcher
object in
CarparkFinderParser
splits the command text into command word and
arguments, in which the command word is checked. Because it is an ambiguous abbrevation
(we do not know if it stands for clear
or calculate
), it is rejected by throwing a ParseException
Step 2. The user now tries s
command instead of select
. The Matcher
object in
CarparkFinderParser
again splits the command text into command word and
arguments, in which the command word is checked. This time, it is checked that the input string
is contained in one of the command words that is calculate
. Therefore, it proceeds as if a
select
command is given.
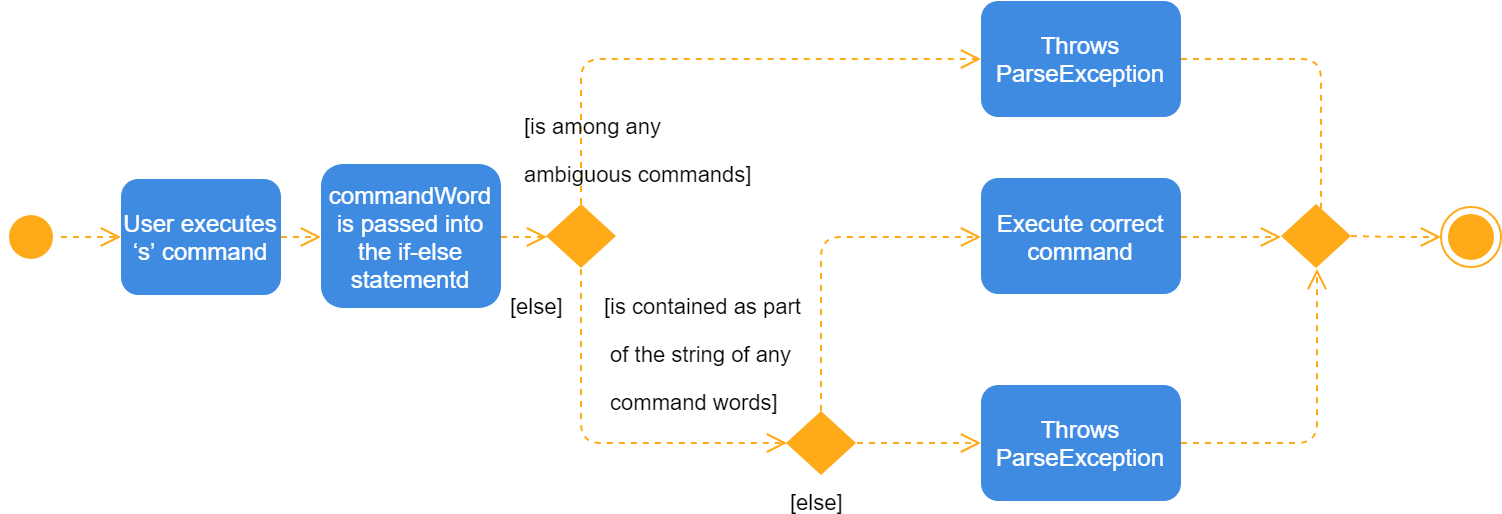
The Activity Diagram above explains what happens when a user executes a f
command.
5.8.3. Design Considerations
Aspect: How abbreviation executes
-
Alternative 1 (current choice): use input string to check if it is contained in any command word string
Pros
Extra logic needed
Cons
Very short command words that contains ambiguous abbreviation is marginalised in this case. For example, the shortest form of
find
isfin
, which makes not much of a difference. -
Alternative 2: (previous choice):* declare
COMMAND_ABBREVIATION
with a string value in each Command file.Pros
Easy to implement
Cons
Have to decide on each abbreviation subjectively which brings down performance if there are a lot of commands
5.9. Autocomplete
The autocomplete feature simplifies overcomplicated commands by prompting correct format.
5.9.1. Overview
The autocomplete mechanism is facilitated by CommandBox
. It calls autocomplete()
to displayFormat()
if applicable command word is entered or to highlight the next
parameter if full format is already provided in the command box.
5.9.2. Example
Given below is an example usage scenario and how the autocomplete mechanism behaves at each step.
Step 1. The user launches the application for the first time.
Step 2. The user enters fi
in command box and then presses Tab . autoComplete()
compares input
through the list of applicable command words and abbreviations, and
proceeds to displayFormat()
because fi
is an ambiguous COMMAND, it fails with an exception.
Step 3. User tries with fil
again. It passes as part of the autocomplete command filter
thus moves on to highlight its first placeholder, DAY
, in the command line. As seen
from the following diagrams.

fil
is entered.
Step 3. The user replaces DAY
with an actual value, SUN
, and presses 'Tab'
key again. autoComplete()
is called again, but because this time it checks that input
isFilterCommandFormat
, the next placeholder, START_TIME
, is highlighted. Result
is shown in the following diagram.

Step 4. The user continues step 3 until all placeholders are filled up with actual values and then presses Enter to execute this command.
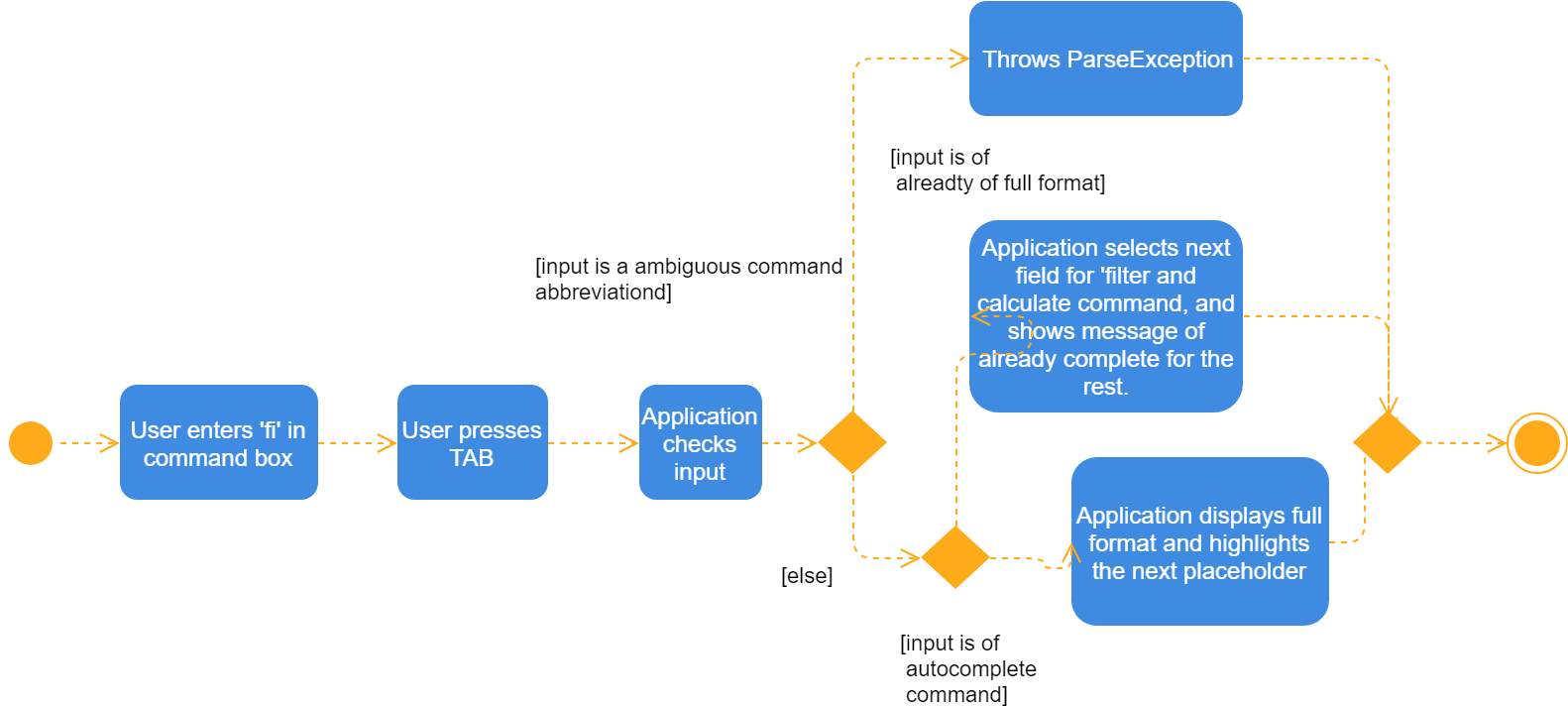
The Activity Diagram above explains what happens when user presses Tab.
5.9.3. Design Considerations
Aspect: TextInput
-
Alternative 1: (current choice): Continue to use the original TextField
Pros
External library enables bindAutocompletion for TextField (the drop down list of suggested commands that appears and updates itself as user types). External library enables bindAutocompletion for TextField (the drop down list of suggested commands that appears and updates itself as user types).
Cons
Text formatting is limited. All text in the TextField must have the same format.
-
Alternative 2: Create additional TextField as user request on additional fields
Pros
Allows for different formatting for different fields (Commands can have a different colour from the fields)
Cons
Original structure will be disrupted. Command box will no longer be single line text input, which have consequences such as the user cannot backspace or select through the entire line.